Introducing filer.js
Some 1300+ lines of code, 106 tests, and a year after I first started it, I’m happy to officially unleash filer.js; a wrapper library for the HTML5 Filesystem API.
Unlike other libraries [1, 2], filer.js takes a different approach and incorporates some lessons I learned while implementing the Google Docs Python client library. Namely, the library reuses familiar UNIX commands (cp
, mv
, rm
) for its API. My goal was to a.) make the HTML5 API more approachable for developers that have done file I/O in other languages, and b.) make repetitive operations (renaming, moving, duplicating) easier.
So, say you wanted to list the files in a given folder. There’s an ls()
for that:
var filer = new Filer();
filer.init({size: 1024 * 1024}, onInit.bind(filer), onError);
function onInit(fs) {
filer.ls('/', function(entries) {
// entries is an Array of file/directories in the root folder.
}, onError);
}
function onError(e) { ... }
A majority of filer.js calls are asynchronous. That’s because the underlying HTML5 API is also asynchronous. However, the library is extremely versatile and tries to be your friend whenever possible. In most cases, callbacks are optional. filer.js is also good at accepting multiple types when working with entries. It accepts entries as string paths, filesystem: URLs, or as the FileEntry
/DirectoryEntry
object.
For example, ls()
is happy to take your filesystem: URL or your DirectoryEntry
:
// These will produce the same results.
filer.ls(filer.fs.root.toURL(), function(entries) { ... });
filer.ls(filer.fs.root, function(entries) { ... });
filer.ls('/', function(entries) { ... });
The library clocks in at 24kb (5.6kb compressed). I’ve thrown together a complete sample app to demonstrate most of filer.js’s functionality:
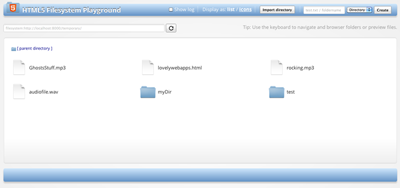
Lastly, there’s room for improvement:
- Incorporate Chrome’s Quota Management API
- Make usage in Web Workers more friendly (there is a synchronous API).
I look forward to your feedback and pull requests!